I’m sorry I told this was not a good idea. My fault. Let’s me recap and say instead: “it’s not usually a good idea”.
But in Frontity people should be able to do pretty much what they want. So if you want to do it because in your case it is a good idea, then yes, this can be done.
You just need to add a handler to access that information.
In this example I am adding a handler for the /wp-json/
endpoint and I am calling it "nameAndDescription"
internally to be able to use it in Frontity.
Once the handler is added, you can use the same actions.source.fetch
and state.source.get
APIs to fetch and use its content.
I have added it only to the server using beforeSSR
and the fetching is done also only in the server. The initial state is sent to the client, so you don’t need to refetch it again in the client. It will be already there.
import Theme from "./components";
import image from "@frontity/html2react/processors/image";
const beforeSSR = async ({ libraries, actions }) => {
// Add image processor.
libraries.html2react.processors.push(image);
// Add the nameAndDescription handler.
libraries.source.handlers.push({
name: "nameAndDescription",
priority: 10,
pattern: "nameAndDescription",
func: async ({ route, state, libraries }) => {
// 1. Get response from api endpoint.
const response = await libraries.source.api.get({
endpoint: "/" // "/" is added after "/wp-json" so final url is "/wp-json/"
});
// 2. Extract relevant data from the response.
const { name, description } = await response.json();
// 3. Add it to the source.data object.
state.source.data[route].name = name;
state.source.data[route].description = description;
}
});
// Fetch the wp-json endpoint.
await actions.source.fetch("nameAndDescription");
};
const beforeCSR = ({ libraries }) => {
// Add image processor.
libraries.html2react.processors.push(image);
};
const marsTheme = {
name: "@frontity/mars-theme",
roots: {
theme: Theme
},
state: {
theme: {
menu: [],
featured: {
showOnList: false,
showOnPost: false
}
}
},
actions: {
theme: {
beforeSSR: beforeSSR,
beforeCSR: beforeCSR
}
}
};
export default marsTheme;
If you inspect frontity.state.source.data
in the client you should see that it is already there:
You can now access it using state.source.get("nameAndDescription")
. Like this:
const Header = ({ state }) => {
const { name, description } = state.source.get("nameAndDescription");
return (
<>
<Container>
<StyledLink link="/">
<Title>{name}</Title>
</StyledLink>
<Description>{description}</Description>
</Container>
<Nav />
</>
);
};
And it should work 
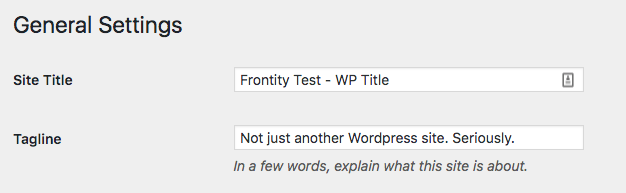
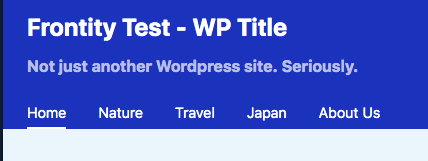