This is a good moment to think again about our eslint
and prettier
configuration so it is as simple as possible.
Eslint
We were following eslint-airbnb
in the past, but I’d like our configuration to be as simple and as standard as possible.
In the past, when prettier
didn’t exist, eslint
did a lot of syntax work. Nowadays much of the work is done by prettier
, so I guess eslint
is less important for syntax. It is still useful to catch errors (no undefined variables and stuff like that).
What happens if we keep only eslint
and jest
?
"extends": [
"eslint:recommended",
"plugin:jest/recommended"
],
I’d like to start as simple as possible, then make things more complex only if we find there’s no other way.
Prettier
I’m thinking we could use prettier with the default config (no config file). Yes, I know, that means no trailing comma, no semis and double quotes 
The main advantage I see is that if people don’t have prettier
well configured, it still works. It also means they don’t need a file in their packages and it also means that it’s the same prettier
config you get in codesandbox by default.
I’m good with whatever you think is better 
Ok, in addition to eslint-plugin-jsx-a11y
(mentioned in Lighthouse Summary), I think we should also use eslint-plugin-react
and eslint-plugin-react-hooks
(not sure if they are already included in the default preset).
And I guess eslint
and prettier
should share the same rules, so one or the other has to adapt, right?
Also, in the previous Frontity we were using also eslint-plugin-import
. Again, I think it was required by some other dependency, but don’t remember exactly which one, maybe airbnb
.
Yes, eslint-plugin-import
was required by airbnb
.
Ok, what about this?
"extends": [
"eslint:recommended",
"plugin:react/recommended",
"plugin:jest/recommended",
"plugin:jsx-a11y/recommended",
"plugin:react-hooks/recommended"
],
react-hooks
doesn’t have a recommended preset yet, but there’s a PR for that:
We can add it when the PR is merged, that way we don’t need to maintain the rules.
Not eslint-plugin-import
?
Hum, I think we are missing typescript-eslint
.
Typescript has its own linter, ts-lint but they are going to join the projects:
But I don’t know much about that really…
Ok, that one was useful, wasn’t it?
Yep, sometimes imports can be a bit tricky.
Ok, this is what they are saying in the TypeScript roadmap:
[…] our editor team will be focusing on leveraging ESLint rather than duplicating work. For scenarios that ESLint currently doesn’t cover (e.g. semantic linting or program-wide linting), we’ll be working on sending contributions to bring ESLint’s TypeScript support to parity with TSLint. As an initial testbed of how this works in practice, we’ll be switching the TypeScript repository over to using ESLint, and sending any new rules upstream.
There is a conflict between @typescript-eslint
and prettier
. Prettier will set an indentation of 2 spaces, while @typescript-eslint
wants 4 spaces.
So we should add
"rules": {
"@typescript-eslint/indent": "off"
}
to our .eslintrc
or change prettier
config. What do you prefer?
eslint
because we already have a .eslintrc
file and if not, we need to add a .prettierrc
file just for that 
There’s a problem with our approach of not using a file for prettier: the vs-code extension uses the default settings each user has defined 
So I guess we need to tell it to be quiet…
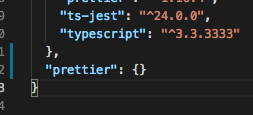
The "prettier": {}
line works fine.
Do you prefer that line in package.json
than {}
in a .prettierrc
file?